Preview 2 of the WCF REST Starter Kit has been posted to CodePlex. Check it out at http://tinyurl.com/wcf-rest-kit2. Included in this latest release of the WCF REST Starter Kit are a great new set of client connection tools that make consumption of RESTful services a breeze.
At the forefront of these client connection tools is the HttpClient. This class allows quick and easy access to download any content from a url. I’ve created a simple WPF Application that demonstrates using the HttpClient and the Live Search API. For more information on the LiveSearch API or to get your LiveSearch API Key goto http://dev.live.com/getstarted/
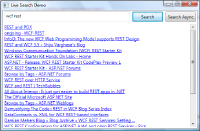
Click here to Download the Full Source Code
Windows Live Search WPF Application
This WPF Application consists of a Window with some code-behind, and a LiveSearch.cs file that was created using the Paste as XML Type feature of the WCF REST Starter Kit. Before you can serialize your results from the Live Search API you need to run the query in your browser and copy the xml results into your clipboard. After that you can go into Visual Studio and create a file in your project called LiveSearch.cs and then go to Edit-> Paste as XML Type. As for the WPF Window it has a Grid with 2 rows and 3 columns. In the top row is a search textbox and two buttons. In the second row is a ScrollViewer that contains an ItemsControl.
The first button does a single threaded search call to the Live Api and sets the ItemsSource of the ItemsControl. As you can see by the code below its very simple, first we construct the uri, then we call .Get on that uri. The Get call returns an HttpResponseMessage. The response message has a number of different chaining methods and extension methods, we are using the EnsureStatusIsSuccess, which is equivalent to the EnsureStatusIs(HttpStatusCode.OK). After we call EnsureStatusIsSuccessful (which returns the original message or an exception) we can read the content. In this case we are reading the content as a xml serialized response of the type created above using the Paste as XML Type feature.
private void Search_Click(object sender, RoutedEventArgs e) { string liveApi = "Your Live Api"; string uriFormat = "http://api.search.live.net/xml.aspx?AppId={0}&Market=en-US&Query={1}&Sources=web&Web.Count=25"; using (var client = new HttpClient()) { string uri = string.Format(uriFormat, liveApi, this.SearchText.Text); var response = client.Get(uri).EnsureStatusIsSuccessful() .Content.ReadAsXmlSerializable<searchResponse>(); this.Results.ItemsSource = response.Web.Results; } }
Since its not recommended to run potentially long running operation on the UI thread. I created a second button to demonstate the Live Search feature using the SendAsync method. Below is the code for that.
private void SearchAsync_Click(object sender, RoutedEventArgs e) { string liveApi = "Your Live Api"; string uriFormat = "http://api.search.live.net/xml.aspx?AppId={0}&Market=en-US&Query={1}&Sources=web&Web.Count=25"; var client = new HttpClient(); string uri = string.Format(uriFormat, liveApi, this.SearchText.Text); client.SendCompleted += client_SendCompleted; client.SendAsync(new HttpRequestMessage(HttpMethod.GET.ToString(), uri)); } void client_SendCompleted(object sender, SendCompletedEventArgs e) { var response = e.Response.EnsureStatusIsSuccessful() .Content.ReadAsXmlSerializable<searchResponse>(); this.Results.ItemsSource = response.Web.Results; }
Feel free to download the full demo using the link above, I hope you enjoy it. The new client connect features of the WCF REST Starter Kit create a really simple way of communicating with third party REST services.
In your example, you don’t get a callback though. Could you elaborate? You are calling async inline. What if you have a div tag that needs to be resfreshed upon completion?