Easily use DesignData with Caliburn.Micro for Windows Store apps
They may have removed the sample data functionality in the latest versions of Blend, but have no fear, you can still accomplish effective Designer/Developer workflows using custom design data and the Model-View-ViewModel (MVVM) Pattern. I was setting up the sample data functionality on our latest mobile project and I wanted to write this quick blog post to help others setting up the same thing. To start I created our Windows Store application, added the Caliburn.Micro NuGet package, and created the initial View and ViewModel for our Hub page.
The first step is to create an extended version of your ViewModel, in my case I created a folder called SampleData and added a new class called HubPageViewModelSampleData.cs
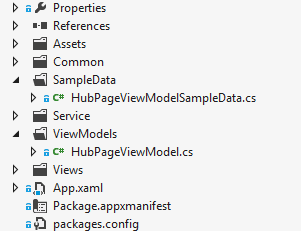
This HubPageViewModelSampleData class inherits from our HubPageViewMode and just initializes the sample data in the constructor.
public class HubPageViewModelSampleData : HubPageViewModel { public HubPageViewModelSampleData() : base(null, null, null) { DisplayName = "Hub"; Sites = new ObservableCollection<Site>() { new Site() { SiteName = "Site 1", SiteUrl = "http://www.example.com/123", Status="Online" }, new Site() { SiteName = "Site 2", SiteUrl = "http://www.example.com/456", Status="Online" }, new Site() { SiteName = "Long Site 1", SiteUrl = "http://www.example.com/123/abcdefg/456/hijklmnop.html", Status="Offline" } }; } }
If your base class has any dependencies injected you can supply a parameterless constructor override with null values or null implementations. You can also insert overridden methods/properties, which I would tend to prefer over injecting your ViewModel with a lot of checks for DesignMode.DesignModeEnabled. Once you have your sample data view model created you just have to wire it up to your view. In order to do this you’ll need setup the design time DataContext with a design time instance to this sample data view model.
<Page x:Class="App.Views.HubPage" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:system="using:System" xmlns:micro="using:Caliburn.Micro" d:DataContext="{d:DesignInstance Type=sampleData:HubPageViewModelSampleData, IsDesignTimeCreatable=True}" mc:Ignorable="d"> <!-- … --> </Page>
Visual Studio supports this design context for DataBinding and will work if you use declarative {Binding} statements in your XAML. While this way doesn’t use Blend and it is still part of your deployed Appx, it is easy to setup. Unfortunately this won’t work with our project because we are using Caliburn.Micro. One of the great features of Caliburn.Micro is the ability to do convention based binding. This feature doesn’t use the declartive Binding syntax that the XAML designer is expecting. Because of this the bindings don’t work with the XAML for a Caliburn.Micro view. Luckily the team has provided an attached property that executes just the ViewBinding portion of the framework in design mode. This attached property Bind.AtDesignTime should be added to the same control that has you’re design DataContext as you can see here.
<Page x:Class="App.Views.HubPage" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:system="using:System" xmlns:micro="using:Caliburn.Micro" xmlns:sampleData="using:App.SampleData" d:DataContext="{d:DesignInstance Type=sampleData:HubPageViewModelSampleData, IsDesignTimeCreatable=True}" micro:Bind.AtDesignTime="True" mc:Ignorable="d"> <!-- … --> </Page>
With this in place we can see the effects of our design decisions right in the designer without having to run the app.
By following these steps you can setup sample data support for all your view models hopefully achieve the symbiotic moment you get from an effective developer/designer workflow.